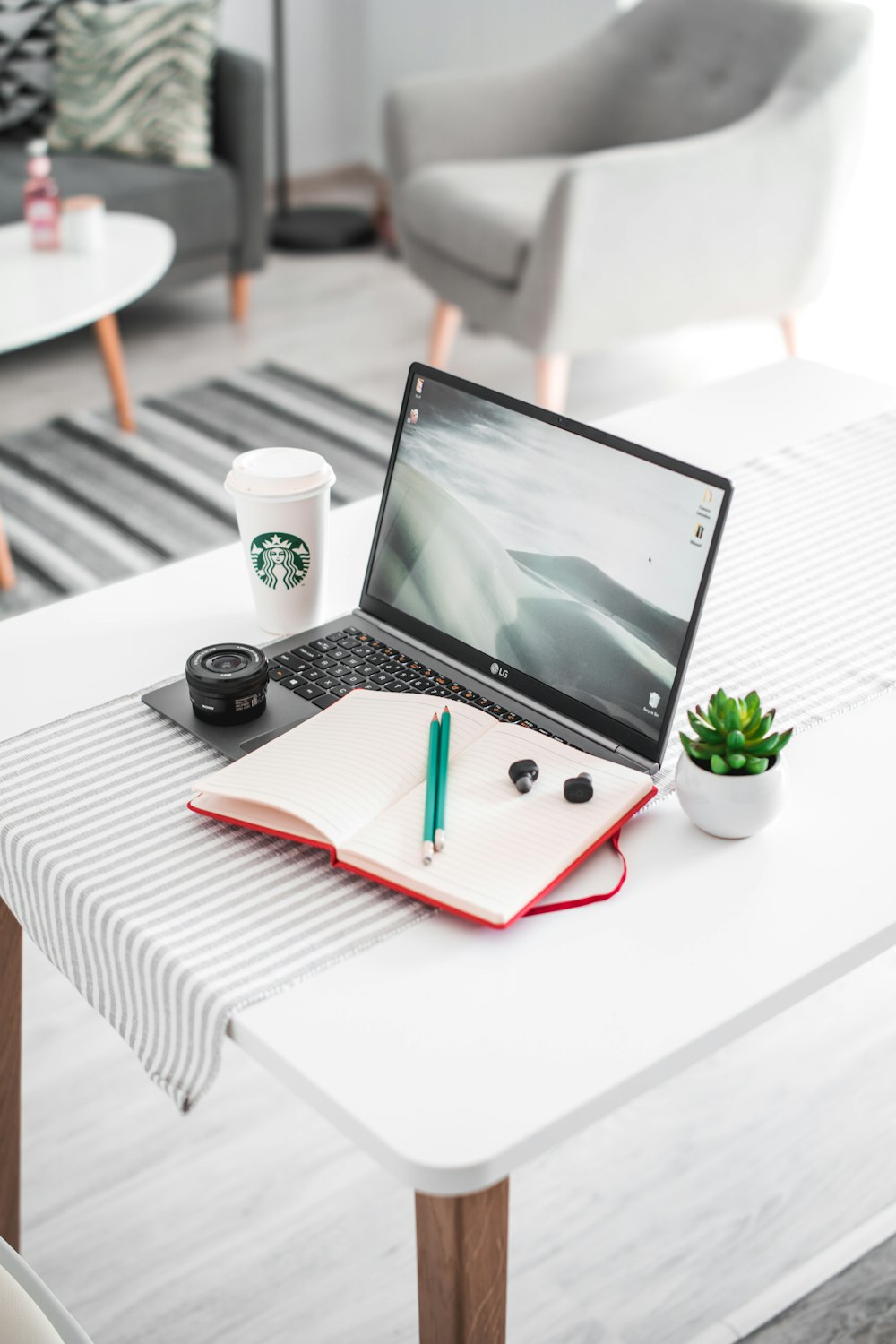
One of the most important features in JavaScript.
Objects and properties
In objects, we define key-value pairs which means each value has a name which is called the key.
Objects are like containers which can store variables called properties.
One fundamental differences between an object and an array is that in array order matters a lot while in object it does not matter at all.
Steps
- Declare a variable
var john =
- The easiest way to create a object is the so called object literal.
var john = {};
- Start defining key-value pairs. In this case, name is the key and ‘john’ is the value. So we call it a key-value pair. We also say that firstName is a property of the john object. We separate properties using coma just like an array.
We can put all types of data types here, such as an array or even place an object inside another.
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false
};
console.log(john);
firstName, lastName, birthYear, familyMembers, job, isMarried are all properties of john object, so how can we access these properties?
Methods to read/ access object’s properties = retrieve data from an object
- Dot notation
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false
};
console.log(john.firstName);
//john
- Use the [key name]
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false
};
console.log(john['lastName']);
//Smith
We have to use single quote as key names are actually strings
or
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false
};
var x = 'birthYear';
console.log(john[x]);
//1998
Object Mutations
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false
};
john.job = 'designer';
john['isMarried'] = true;
console.log(john);
//{firstName: "john", lastName: "Smith", birthYear: 1998, familyMembers: Array(3), job: "designer", …}birthYear: 1998familyMembers: (3) ["Jane", "Mark", "Emily"]firstName: "john"isMarried: truejob: "designer"lastName: "Smith"__proto__: Object
There is another to initialize an object.
var jane = new Object();
jane.name = 'Jane';
jane.birthYear = 1988;
jane['lastName'] = 'Smith';
console.log(jane);
In summary, there are two ways to create objects.
- Object literal { }
- new Object() syntax
Methods
Objects can hold different types of data, including arrays, objects etc.
In fact, we can also attach functions to objects, and these functions are called methods.
To create a method for an object, firstly
- Define the key
- Define the value, and the value here is basically a function expression where the function doesn’t have a name, we pass in an argument and later we assign this function to a variable which in this case called calculateAge.
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false,
calculateAge: function(birthYear){
return 2018 - birthYear;
}
};
The way we call the function is the same as accessing the properties and normal function calls.
console.log(john.calculateAge(1998));
//20
Recall that in the previous note we reviewed some methods that can be applied to arrays.
In fact, arrays are also objects and that’s the only way they can have methods.
Only objects can have methods.
However, the age (1998) that we pass in as the argument is already defined in the object itself.
Hence, instead of passing it to the method again, how can we access the birthYear property right inside this object ?
In every object, JavaScript gave us a special keyword called this.
Therefore, instead of passing birthYear again to the calculatedAge, we can simply say
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false,
calculateAge: function(){
return 2018 - this.birthYear;
}
};
console.log(john.calculateAge());
//20
‘this’ in this case refers to the john object.
So basically,
‘this’ means the current object.
return 2018 - this.birthYear == 2018 - john.birthYear
Ok, so what if we want to store this result into the john object ?
var john = {
firstName: 'john',
lastName: 'Smith',
birthYear: 1998,
familyMembers: ['Jane', 'Mark', 'Emily'],
job: 'teacher',
isMarried: false,
calculateAge: function(){
this.age = 2018 - this.birthYear;
}
};
john.calculateAge();
console.log(john);
//
age: 20
birthYear: 1998
calculateAge: ƒ ()
familyMembers: (3) ["Jane", "Mark", "Emily"]
firstName: "john"
isMarried: false
job: "teacher"
lastName: "Smith"
__proto__: Object
we can also set/add a property to an object using ‘this’ just like the case above.
this.age = 2018 - this.birthYear;
//age: 20
Please note that this is my study notes that I took while taking the complete guide to JavaScript course on Udemy, if you are interested in understanding the language better, I highly recommend you to purchase the course 🙂 !